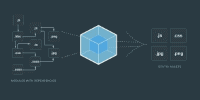
Webpack多页面多入口配置
一般来说,Webpack都是用来打包单页面应用的,只有一个首页和入口文件,但是在写传统的Html页面时候,还是需要些很多的页面和很多个入口,这时候就需要对Webpack进行不一样的配置了,下面是我自己的一些摸索。下面是我的webpack.config.js
的具体配置:
const path = require('path');
const webpack = require('webpack');
const UglifyJsPlugin = require('uglifyjs-webpack-plugin');
const ExtractTextPlugin = require('extract-text-webpack-plugin'); //分离CSS和JS文件
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
entry: {
bundle1: './app/js/index1.js',
bundle2: './app/js/index2.js',
common: ['jquery']
},
output: {
path: path.resolve(__dirname, './build'),
//publicPath:"/assets/",
filename: '[name].js'
},
module: {
rules: [
{
test: /\.less$/,
use: ExtractTextPlugin.extract({
fallback: "style-loader",
use: [{
loader: 'css-loader',
options:{
minimize: true //css压缩
}
},
"less-loader"
]
})
},
{
test: /(\.jsx|\.js)$/,
use: {
loader: "babel-loader"
},
exclude: /node_modules/
},
{
//html模板加载器,可以处理引用的静态资源,默认配置参数attrs=img:src,处理图片的src引用的资源
//比如你配置,attrs:[img:src, img:data-src]就可以一并处理data-src引用的资源了,就像下面这样
test: /\.html$/,
use: {
loader: 'html-loader',
options: {
attrs: ['img:src','img:data-src']
}
}
},
{
test: /\.(woff|woff2|ttf|eot|svg)(\?v=[0-9]\.[0-9]\.[0-9])?$/,
use:["file-loader"]
},
{
test: /\.(png|jpg|gif)$/,
use: [{
loader: 'url-loader',
options: {
limit: 8192
}
}]
}
]
},
devtool: 'cheap-module-source-map', //生成Source Maps(使调试更容易)
//使用webpack-dev-server
devServer: {
historyApiFallback: true,
contentBase: './dist',
host: '127.0.0.1',
progress: true, //报错无法识别,删除后也能正常刷新
port: 8080,
inline: true,
hot: true
},
plugins: [
new UglifyJsPlugin(), //压缩代码
new ExtractTextPlugin({ //css提取为单独的文件
filename: '[name].css'
}),
new CommonsChunkPlugin({
name: "chunk", //打包公共代码
minChunks: 2
}),
new CommonsChunkPlugin({
name: "common", //打包公共代码
minChunks: Infinity
}),
new HtmlWebpackPlugin({
//title: 'test',
inject: 'head',
favicon: '',
minify: { //压缩HTML文件
removeComments: true, //移除HTML中的注释
collapseWhitespace: true //删除空白符与换行符
},
hash: true, //为静态资源生成hash值
filename: 'index1.html',
chunks: ['bundle1'] , //需要引入的chunk,不配置就会引入所有页面的资源
template: __dirname + "/app/html/index1.html"//new 一个这个插件的实例,并传入相关的参数
}),
new HtmlWebpackPlugin({
filename: 'index2.html',
chunks: ['bundle2'] ,
template: __dirname + "/app/html/index2.html"//new 一个这个插件的实例,并传入相关的参数
})
]
};
```
## 需要注意的点
- #### `html-loader`
由于是传统的Html页面,不像单页面应用是`jsx`语法,DOM元素写在html里,如果加载静态资源的话,就无法进行路径的改写,而html-loader就很好的解决了这个问题,它会根据你的配置,把元素里面的属性(一般是src)进行路径改写成打包后的正确路径。
- #### `html-webpack-plugin`
由于页面比较多,所以模板也比较多,所以要调用很多次
```js
new HtmlWebpackPlugin()
```
如果觉得这样写太过麻烦,也可以这样写
```js
//根据首页列表数据生成很多个文章内容页面
const HtmlArray = [1,2,3,4,5,6,7,8,9,10];
HtmlArray.forEach((item, index) => {
const newPlugin = new HtmlWebpackPlugin({
filename: 'article'+index + '.html',
template: __dirname + "/app/html/article.html",
inject: 'head', // 插入文件的位置
hash: true, // 在生成的文件后面增加一个hash,防止缓存
minify: { //压缩HTML文件
removeComments: true, //移除HTML中的注释
collapseWhitespace: true //删除空白符与换行符
},
chunks: ['common','bundle1']
});
module.exports.plugins.push(newPlugin);
```