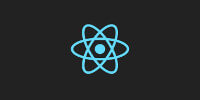
react使用中的一些经验和总结
前言
最近一直在用React,在使用的过程中也遇到了很多的问题,然后通过思考,解决问题,也进一步加深了对React的理解,现在我就简单的总结一下自己遇到的问题和思考出的经验。
React生命周期图
componentWillReceiveProps
- 父组件只要重新渲染就会触发子组件的componentWillReceiveProps,如果属性没有变化的话,不会触发子组件的render
- componentwillreceiveprops在初始化的时候是不会执行的
- componentwillreceiveprops中nextprops和this.props不一样,在shouldcomponentupdate之后才会一致
事件写法
在React中写事件执行的方法的时候。有3种方法可以绑定this对象:
- 使用回调函数方法
handleClick() {
console.log('this is:', this);
}
render() {
return (
<button onClick={(e) => this.handleClick(e)}>
Click me
</button>
);
}
- 使用bind方法
handleClick() {
console.log('this is:', this);
}
render() {
return (
<button onClick={this.handleClick.bind(this)}>
Click me
</button>
);
}
- 使用箭头函数在定义时候就绑定this对象
handleClick = ()=> {
console.log('this is:', this);
}
render() {
return (
<button onClick={this.handleClick}>
Click me
</button>
);
}
state
state写与不写在constructor中没有什么区别,如果是需要根据初始化时传入的props来计算出state的话,则需要写在constructor中
defaultProps
与propTypes
定义组件的属性类型和默认属性
在ES6里,可以统一使用static成员来实现
class Video extends React.Component {
static defaultProps = {
}
static propTypes = {
}
}
也可以写在class外面:
class Video extends React.Component {
}
Video.defaultProps = {
};
Video.propTypes = {
};
getInitialState
与getDefaultProps
这两个方法是在Es5版本的React中才有,在ES6中可以直接设置state和defaultProps
shouldComponentUpdate
在shouldcomponentupdate不要对state进行操作,如果需要的话,可以在componentwillreceiveprops进行设置
componentwillunmout
componentwillunmout会在子组件不在父组件的render的React的Dom树中出现时候执行,例如:
state = {e: 0}
onChange = (ev) => {
console.log(ev.target.value)
this.setState({
e: ev.target.value
})
}
render(){
const { e } = this.state;
return(
<article>
<input value={e} type="text" onChange={this.onChange}/>
{ (e === 0) ? <A /> : <p>sss</p> }
</article>
)
}
只要e不为0的时候,就会触发A组件的componentwillunmout
与第三方JS插件集成
如果是一些传入DOM节点的插件,可以直接使用ref,但是如果是一些传入ID的,情况就有点特殊了,因为react如果复用组件的时候,生成的dom会有多个实例,所以如果集成一些需要对ID进行操作的js代码或者框架,需要将ID设置为变量,也就是需要为每个调用设置成不同的ID
解构&属性延展
结合使用ES6+的解构和属性延展,我们给子组件传递一批属性更为方便了。这个例子把className以外的所有属性传递给div标签:
render() {
var {
className,
...others
} = this.props;
return (
<div className={className}>
<Child {...others} />
</div>
);
}
下面这种写法,则是传递所有属性的同时,用覆盖新的className值:
<div {...this.props} className="override">
</div>
这个例子则相反,如果属性中没有包含className,则提供默认的值,而如果属性中已经包含了,则使用属性中的值
<div className="base" {...this.props}>
</div>