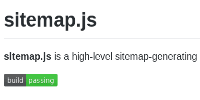
Nodejs使用sitemap库生成网站地图
简介
sitemap.js是一个高级的网站地图生成框架,可以轻松地创建站点地图XML文件。
github地址是https://github.com/ekalinin/sitemap.js
安装
推荐使用npm进行安装
npm install --save sitemap
使用
var sm = require('sitemap')
// Creates a sitemap object given the input configuration with URLs
var sitemap = sm.createSitemap({ options });
// Generates XML with a callback function
sitemap.toXML( function(err, xml){ if (!err){ console.log(xml) } });
// Gives you a string containing the XML data
var xml = sitemap.toString();
//options 包含三个基本参数
hostname: 'http://example.com', //主机名
cacheTime: 600000, // 缓存时间
urls: [ //要生成的网站地图的url
{ url: '/page-1/', changefreq: 'daily', priority: 0.3 },
{ url: '/page-2/', changefreq: 'monthly', priority: 0.7 },
{ url: '/page-3/' } // changefreq: 'weekly', priority: 0.5
]
//其他用法
sitemap.toString() //将网站地图生成字符串形式
sitemap.add({url: '/page-2/', changefreq: 'monthly', priority: 0.7}); //添加一个url
sitemap.del({url: '/page-2/'}); //删除一个url
例子
- 配合express使用
var express = require('express'),
sm = require('sitemap');
var app = express(),
sitemap = sm.createSitemap ({
hostname: 'http://example.com',
cacheTime: 600000, // 600 sec - cache purge period
urls: [
{ url: '/page-1/', changefreq: 'daily', priority: 0.3 },
{ url: '/page-2/', changefreq: 'monthly', priority: 0.7 },
{ url: '/page-3/'}, // changefreq: 'weekly', priority: 0.5
{ url: '/page-4/', img: "http://urlTest.com" }
]
});
app.get('/sitemap.xml', function(req, res) {
sitemap.toXML( function (err, xml) {
if (err) {
return res.status(500).end();
}
res.header('Content-Type', 'application/xml');
res.send( xml );
});
});
app.listen(3000);
- 在静态文件基础上提前生成sitemap
var sm = require('sitemap'),
fs = require('fs');
var sitemap = sm.createSitemap({
hostname: 'http://www.mywebsite.com',
cacheTime: 600000, //600 sec (10 min) cache purge period
urls: [
{ url: '/' , changefreq: 'weekly', priority: 0.8, lastmodrealtime: true, lastmodfile: 'app/assets/index.html' },
{ url: '/page1', changefreq: 'weekly', priority: 0.8, lastmodrealtime: true, lastmodfile: 'app/assets/page1.html' },
{ url: '/page2' , changefreq: 'weekly', priority: 0.8, lastmodrealtime: true, lastmodfile: 'app/templates/page2.hbs' } /* useful to monitor template content files instead of generated static files */
]
});
fs.writeFileSync("app/assets/sitemap.xml", sitemap.toString());
- 给图片加上标题
var sitemap = sm.createSitemap({
urls: [{
url: 'http://test.com/page-1/',
img: [
{
url: 'http://test.com/img1.jpg',
caption: 'An image',
title: 'The Title of Image One',
geoLocation: 'London, United Kingdom',
license: 'https://creativecommons.org/licenses/by/4.0/'
},
{
url: 'http://test.com/img2.jpg',
caption: 'Another image',
title: 'The Title of Image Two',
geoLocation: 'London, United Kingdom',
license: 'https://creativecommons.org/licenses/by/4.0/'
}
]
}]
});
- 视频
var sitemap = sm.createSitemap({
urls: [{
url: 'http://test.com/page-1/',
video: [
{ thumbnail_loc: 'http://test.com/tmbn1.jpg', title: 'A video title', description: 'This is a video' },
{ thumbnail_loc: 'http://test.com/tmbn2.jpg', title: 'Another video title', description: 'This is another video' },
]
}]
});
- 给动态网页添加进sitemap
var sitemap = sm.createSitemap ({
hostname: 'http://example.com',
cacheTime: 600000
});
sitemap.add({url: '/page-1/'});
sitemap.add({url: '/page-2/', changefreq: 'monthly', priority: 0.7});
sitemap.del({url: '/page-2/'});
sitemap.del('/page-1/');